Implementation of OAuth 2.0 | Step-by-Step Guide 🆕
Before digging into the required steps to make your application work under oAuth 2.0, make sure you are familiarised with the flow used at Brevo. For this guide we will dive into a practical example on Node.js. We will create a service running in localhost which handles the Brevo auth server callbacks. This should be able to give you a clear idea of the flow in runtime.
Your access credentials
Once you have completed submitting your app and the approval process is completed you should receive the following credentials. Think of these as a username:password pair for your application, if you wish. They should never be hardcoded in client facing code otherwise any developer can impersonate your application.
Item | Description | Example |
---|---|---|
client_id | This is the name id of the project you have submitted to us. | my-new-app |
client_secret | This is the token which will be used to authenticate your users. We are able to map any actions between your app and our API with this item. | 3czT4bbqfmwN452B2VrqzO2iwTlJ452c |
code | This is the token retrieved from the Brevo auth server every time an end user authenticates with your application. Is a JWT based token | ab5a58c3-8ade-49ff-8f3c-e068548a478c.47a7dcff-3b84-43f4-8b32-99a9417896ba.5896f387-e86d-455d-a918-2fb8e8907936 |
Create a redirection service
Create with express a simple service which receives the callback from the Brevo auth server. This will be the callback URL you submitted in your application form.
const express = require('express');
const app = express();
const port = 3000;
app.get('/redirect', (req, res) => {
// Here we will print the access_code retrieved from the Brevo auth Server.
console.log(JSON.stringify(req.query.code));
//We will also return all the paramaters available in the callback.
res.json(JSON.stringify(req.query));
});
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Define your login route
This is the URL path that you will distribute among your users to allow them to authenticate into Brevo. This URL has two query parameters which you need to modify:
client_id
: This will be the name we have assigned to your OAuth application.redirect_uri
: This will be the URI that you have defined for handling the redirect event from Brevo auth server.
https://auth.brevo.com/realms/apiv3/protocol/openid-connect/auth?response_type=code&client_id={{YOUR_CLIENT_ID}}&redirect_uri={{YOUR_CALLBACK_URL}}&scope=openid
This url will render a login screen . Note that the above URL will be converted to an encoded version not to expose your variables. It will look something like this.
https://auth.brevo.com/realms/apiv3/login-actions/authenticate?execution=8801c140-bd81-4f06-3251-8a5c0f54e7f9&client_id={{YOUR_CLIENT_ID}}&tab_id=e0h0VvmWuJM
End-user authentication and callback
Once your end user has succesfully authenticated on Brevo. The Brevo auth server will execute the callback action to your provided redirect_uri
and return there the full payload from the Brevo auth server. You will see something like this.
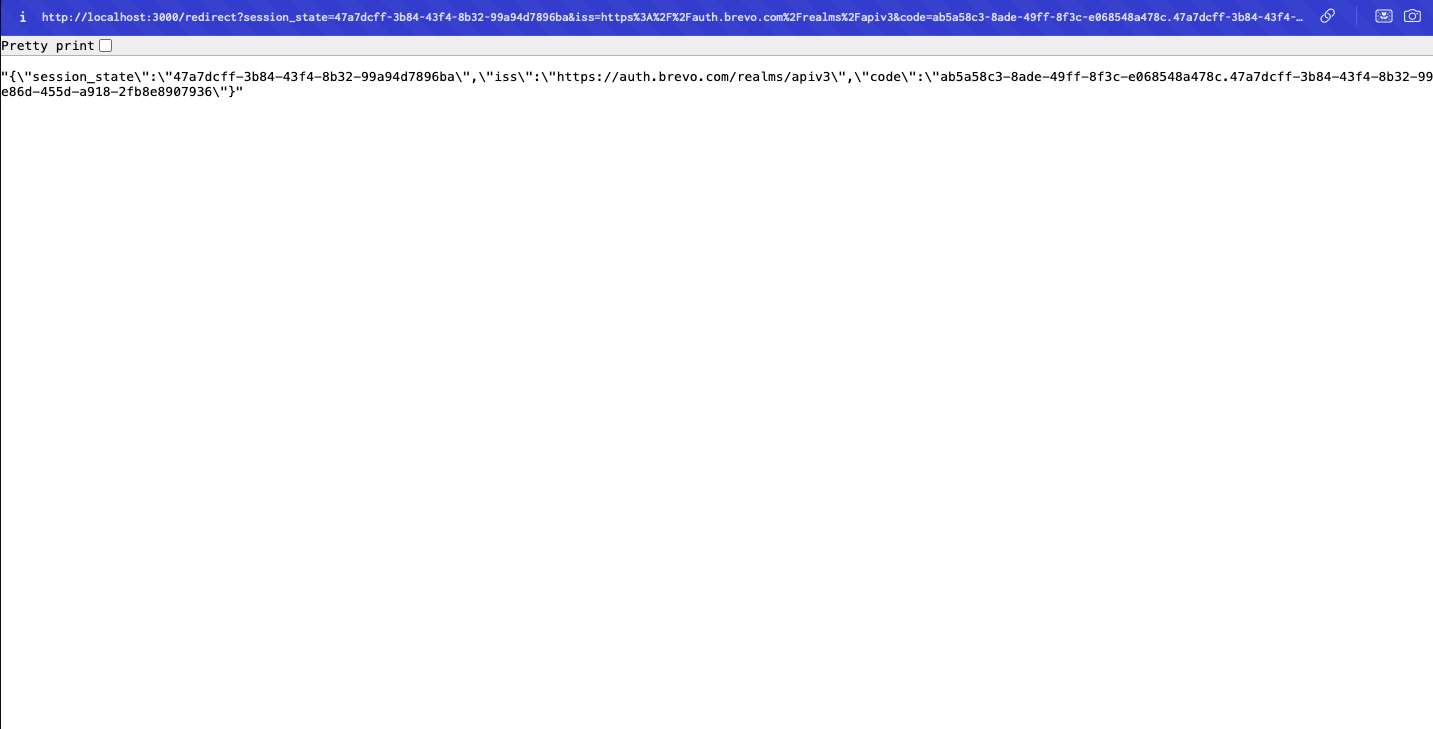
In your running express app @ http://localhost:3000
. You should be able to see the value for code
printed in the console. Note that the TTL of code
is by default 10 minutes.
Fetching your access-token
Now that you finally have your client_id
, app_secret
and code
you are all set for using oAuth to interface with Brevos APIs. Last step is to get your bearer token (access_token
) , this is the Authorization header you need to include for all API calls. In this step we will call the token (https://api.brevo.com/v3/token
) endpoint to retrieve the access-token
curl --location 'https://api.brevo.com/v3/token' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=authorization_code' \
--data-urlencode 'client_id={{YOUR_CLIENT_ID}}' \
--data-urlencode 'client_secret={{YOUR_CLIENT_SECRET}}' \
--data-urlencode 'code={{YOUR_CODE}}' \
--data-urlencode 'redirect_uri=http://localhost:3000/redirect'
After calling this endpoint, you will receive the following payload with the final OAuth credentials.
{
"access_token":"eyJhbGciOiJSU426234IsInR5cCIgOiAiSldUIiwia2lkIiA6ICJfb0pRamM0Q1EzaVByUUxTcWk2TEZmNGp6c0ZFdzlkWmhXaVVHMkExYkJnIn0.eyJleHAiOjE3MTk2MDk4MDAsImlhdCI6MTcxOTU2NjYwMCwiYXV0aF90aW1lIjoxNzE5NTY1NTE1LCJqdGkiOiJkY2M3OGRjYy1kYzViLTRlMmMtOWI1MS05NGZiOGZjMjM2YzAiLCJpc3MiOiJodHRwczovL2F1dGguYnJldm8uY29tL3JlYWxtcy9hcGl2MyIsImF1ZCI6ImFjY291bnQiLCJzdWIiOiI1ZmM5Y2Y5MS01ODI5LTQyNDctODQwOS1lNjhhYjFmNTQ1MTYiLCJ0eXAiOiJCZWFyZXIiLCJhenAiOiJlbnZveS1hdXRoIiwic2Vzc2lvbl9zdGF0ZSI6IjQ3YTdkY2ZmLTNiODQtNDNmNC04YjMyLTk5YTk0ZDc4OTZiYSIsImFjciI6IjAiLCJhbGxvd2VkLW9yaWdpbnMiOlsiKiJdLCJyZWFsbV9hY2Nlc3MiOnsicm9sZXMiOlsiZGVmYXVsdC1yb2xlcy1hcGl2MyIsIm9mZmxpbmVfYWNjZXNzIiwidW1hX2F1dGhvcml6YXRpb24iXX0sInJlc291cmNlX2FjY2VzcyI6eyJhY2NvdW50Ijp7InJvbGVzIjpbIm1hbmFnZS1hY2NvdW50IiwibWFuYWdlLWFjY291bnQtbGlua3MiLCJ2aWV3LXByb2ZpbGUiXX19LCJzY29wZSI6Im9wZW5pZCBlbWFpbCBtZXRhSW5mbyBwcm9maWxlIiwic2lkIjoiNDdhN2RjZmYtM2I4NC00M2Y0LThiMzItOTlhOTRkNzg5NmJhIiwiZW1haWxfdmVyaWZpZWQiOmZhbHNlLCJuYW1lIjoiTWF1cmljaW8gTW91cnJhaWxsZSIsInByZWZlcnJlZF91c2VybmFtZSI6Im1hdXJpY2lvQGJyZXZvLmNvbSIsImdpdmVuX25hbWUiOiJNYXVyaWNpbyIsImxvY2FsZSI6ImVuIiwiZmFtaWx5X25hbWUiOiJNb3VycmFpbGxlIiwiZW1haWwiOiJtYXVyaWNpb0BicmV2by5jb20ifQ.pROVoMSOGgbK88lbsHHUP2RtGRFOfmPIWCxI9UhErAm5Juu-PSGNI9sF4c52oPQjF2lx7Y3Nq8gc6jXE94T7WIS3hwRG0-saIJjqqTvYJnZ58PQXvkpRByezE6UPYpLUBdevpxyWSZV05OHGuMH6BYGmSYFoM92N8EtbPPaCzriPIoERhAKenDuO_E-7ZpxJGBwWDGOHkfj9O-fFwp-_ITuuID9bkp4kA7c5ctJ__k_Ll4MxxuoF3LZUyVGVPaXmZFOqTASQVzoV6nBm4vZNSA4qyqNno6DXAe1NpIHtcmPjPkk4LQgr38L7glXJtd47bZkdBqj0JGCUYuz2u01jLA",
"expires_in":43200,
"refresh_expires_in":31534915,
"refresh_token":"eyJhbGciOiJIUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJiY2E0ODRjYS01ZTFmLTRiZDctYjM4NC1hNjA1YjhlZjAzZWYifQ.eyJleHAiOjE3NTExMDE1MTUsImlhdCI6MTcxOTU2NjYwMCwianRpIjoiZmM3Njg2NzMtODU3OC00MmE1LWJhZjMtY2UwZWJjNzVmOTcyIiwiaXNzIjoiaHR0cHM6Ly9hdXRoLmJyZXZvLmNvbS9yZWFsbXMvYXBpdjMiLCJhdWQiOiJodHRwczovL2F1dGguYnJldm8uY29tL3JlYWxtcy9hcGl2MyIsInN1YiI6IjVmYzljZjkxLTU4MjktNDI0Ny04NDA5LWU2OGFiMWY1NDUxNiIsInR5cCI6IlJlZnJlc2giLCJhenAiOiJlbnZveS1hdXRoIiwic2Vzc2lvbl9zdGF0ZSI6IjQ3YTdkY2ZmLTNiODQtNDNmNC04YjMyLTk5YTk0ZDc4OTZiYSIsInNjb3BlIjoib3BlbmlkIGVtYWlsIG1ldGFJbmZvIHByb2ZpbGUiLCJzaWQiOiI0N2E3ZGNmZi0zYjg0LTQzZjQtOGIzMi05OWE5NGQ3ODk2YmEifQ.mQLS52E_bYiYLBkYtQWPeGEUaCa8efJJD-wXUAya4rY",
"token_type":"Bearer",
"id_token":"eyJhbGciOiJSUzI1NiIsInR5cCIgOiAiSldUIiwia2lkIiA6ICJfb0pRamM0Q1EzaVByUUxTcWk2TEZmNGp6c0ZFdzlkWmhXaVVHMkExYkJnIn0.eyJleHAiOjE3MTk2MDk4MDAsImlhdCI6MTcxOTU2NjYwMCwiYXV0aF90aW1lIjoxNzE5NTY1NTE1LCJqdGkiOiIzZDA0ZTg4MS1iMmJkLTQxZWItOTc4Ni1lNWQ4OTAxZTdlNTUiLCJpc3MiOiJodHRwczovL2F1dGguYnJldm8uY29tL3JlYWxtcy9hcGl2MyIsImF1ZCI6ImVudm95LWF1dGgiLCJzdWIiOiI1ZmM5Y2Y5MS01ODI5LTQyNDctODQwOS1lNjhhYjFmNTQ1MTYiLCJ0eXAiOiJJRCIsImF6cCI6ImVudm95LWF1dGgiLCJzZXNzaW9uX3N0YXRlIjoiNDdhN2RjZmYtM2I4NC00M2Y0LThiMzItOTlhOTRkNzg5NmJhIiwiYXRfaGFzaCI6IndtVnl2UVdGN1Z5Q3pGZW9DZEU3SHciLCJhY3IiOiIwIiwic2lkIjoiNDdhN2RjZmYtM2I4NC00M2Y0LThiMzItOTlhOTRkNzg5NmJhIiwiZW1haWxfdmVyaWZpZWQiOmZhbHNlLCJuYW1lIjoiTWF1cmljaW8gTW91cnJhaWxsZSIsInByZWZlcnJlZF91c2VybmFtZSI6Im1hdXJpY2lvQGJyZXZvLmNvbSIsImdpdmVuX25hbWUiOiJNYXVyaWNpbyIsImxvY2FsZSI6ImVuIiwiZmFtaWx5X25hbWUiOiJNb3VycmFpbGxlIiwiZW1haWwiOiJtYXVyaWNpb0BicmV2by5jb20ifQ.b44PTMX6BMgTOI5_JyMs-zI3dhnrbJLpHeadta-OdsBGSOA8h4PGRuHLTvqsBEgXcW2OeyDpnpDcYK8GrNxiKP2fEbHaB1bRTNw4s2J_Qsicf27rj3WXQwD0WF1VrKiAIH3It9uuUpRifSlAwkmf-qwoWmHGNnCEGK7leYqALAhVHrvowolP-KG58W4LhqLSrIDsMYR8I25Oy3B8im_IgQv2_xxmsz-ZauiDUzyrpUQWiVbqzK_aENCSQD940CJzS52-W-4ukwguhev7ZnUFPKxVgWjV-I__a10bqX48IXMYN-5MzKwo4rlrqDTCVc6jOjXAY84EOOPd6jCmH9hbRQ",
"session_state":"47a7dcff-3b84-43f4-8b32-99a94d7896ba",
"scope":"openid email metaInfo profile"
}
Calling Brevo APIs with your access-token
You made it, you can finally use OAuth to interact with all of Brevo's APIs in a secure way.
curl --request GET \
--url https://api.brevo.com/v3/account \
--header 'accept: application/json' \
--header 'Authorization: Bearer {{YOUR_ACCESS_TOKEN}}'\
If your access_token
is valid that means you can successfully retrieve your account details, for this example, note you can now use your access token in the above form to interact with all of our endpoints. 🎉
{
"plan": [
{
"type": "subscription",
"credits": 39983,
"creditsType": "sendLimit",
"startDate": "2017-03-11",
"endDate": "2017-04-11"
}
],
"relay": {
"enabled": true,
"data": {
"userName": "[email protected]",
"relay": "smtp-relay.domain.com",
"port": 587
}
},
"marketingAutomation": {
"key": "kzfr5xxxxxxttuyo1",
"enabled": true
},
"email": "[email protected]",
"firstName": "John",
"lastName": "Smith",
"companyName": "MyShop",
"address": {
"city": "New-York",
"street": "1677B 8th Avenue",
"zipCode": "7665",
"country": "USA"
}
}
Updated 10 months ago