EMAIL | Send a transactional message
Learn how to send a transactional email for an existing template and adding dynamic content
Transactional emails are used for all non-promotional emails: send them when a user has created an account, when they have made an order, when request a new password...
What you will learn from this tutorial
In this tutorial, you will learn how to:
send a basic transactional email with a dummy HTML content.
send a transactional email where we'll insert dynamic contact attributes (ex. contact name, address) and transactional parameters (ex. order number, expected date of delivery).
Requirements
Get your API key from your settings (SMTP & API).
If you are new to the API, read more about how the api works.
Send a transactional email using a basic HTML content
Let's assume we would like to send the following basic email.
HTML content | <html><head></head><body><p>Hello,</p>This is my first transactional email sent from Brevo.</p></body></html> |
Email subject | "Hello worldl" |
Sender name | "Sender Alex" |
Sender email | "email":"[email protected]" |
Recipient name | "John Doe" |
Recipient email | "email":"[email protected]" |
The HTTP request to send using cURL is the following:
curl --request POST \
--url https://api.brevo.com/v3/smtp/email \
--header 'accept: application/json' \
--header 'api-key:YOUR_API_KEY' \
--header 'content-type: application/json' \
--data '{
"sender":{
"name":"Sender Alex",
"email":"[email protected]"
},
"to":[
{
"email":"[email protected]",
"name":"John Doe"
}
],
"subject":"Hello world",
"htmlContent":"<html><head></head><body><p>Hello,</p>This is my first transactional email sent from Brevo.</p></body></html>"
}'
Send a transactional email containing dynamic contact attributes and transactional parameters
In this tutorial, we'll be sending an order confirmation email to a contact, where we'll insert dynamic contact attributes (contact name, address) and transactional parameters (order number, expected date of delivery).
For the sake of simplicity, we'll use an existing email template to design our email.
This is the email you should get by the end of the tutorial:
Create a test template that includes dynamic content
To get started quickly, let's create the email template from Brevo dashboard.
- First, go to the template creation page
- Choose your settings and click Next Step
- Under Template Name, enter "Order confirmation"
- Under Subject Line, enter "Your new order has been received"
- Pick an existing sender for the From Email
- Enter your company name under From Name
- Once directed the Design tab, select the "Import a template" tab, and copy and paste the following Shared Template URL:
https://my.brevo.com/iNF7GRiE34wzuyVRWobyyLLNbttWwwKn8CQLDhU7kui0HXu4tZG_ZzZ6Ng--
- Click "Import" next to the URL. You should be redirected to the following page bellow.
Click the "Save & Quit" button, then on the new page " Save and Activate".
- Go back to the templates page and save the template id of the one you just created for later. The id of the template is the number after the "#" after the template name.
Template creation using the API
Creating a template using the API is possible, but more limited. Using the Create an smtp template endpoint, you can create a template:
- by passing the html content as a string (
htmlContent
)- by passing the html content available at a URL (
htmlUrl
)
Add a contact attribute for the delivery address
By default, a contact comes with the attributes EMAIL
, FIRSTNAME
, LASTNAME
, SMS
. Let's add the DELIVERYADDRESS
so we can use it fill our template when sending an email.
- Go to the contact attributes page (under Contact > Settings > Contact Attributes & CRM) and add a new field DELIVERYADDRESS.
- Go to your contact page and add a new contact:
FIRSTNAME:
John
LASTNAME:Doe
EMAIL: set up your email to receive the test email
DELIVERYADDRESS:75014 Paris, France
Select any contact list you want to import this contact into
- Click " Save and Close".
Managing contacts
💡 These features are also available through the API. The endpoints that will help you are Creates contact attribute and Create a contact. You can also follow the tutorial on how to synchronise contact lists to be guided through the steps.
👍 That's it! Now let's send our first email!
Send your transactional email
Let's learn how to use the API. The best way to know what's possible and how to use each endpoint is to go to the API Reference.
Under SMTP > Send a transactional email, you can find the description of the endpoint:
1. Generate a code snippet to quickly test your request:
- Enter the body and path parameters to send in your request. These are the ones we'll need:
sender
: Enter your sender email and name
💡 the sender email must be a sender registered and verified in Brevo
💡 the sendername
parameter here can override the default sender name you have setto
: Enter your recipient email and name
💡 the recipient email should be a contact registered in Brevo and assigned to a contact list. That contact should have the attributesFIRSTNAME
,LASTNAME
,EMAIL
,DELIVERYADDRESS
defined.
💡 the recipient name here is the name that will be attached to the email recipient. It will appear in the email headers/metadata and not in the email body.templateId
: Enter your template id
Get the list from the templates page or using the endpoint to get the list of SMTP templatesparams
: the value we will use is{"ORDER": 12345, "DATE": "12/06/2019"}
- Try out the endpoint from the interface. For that, click the " Try it" button and enter you API key
- If everything goes well, you should get a success response (code 201) with a JSON body giving you the id of the message sent. ex:
{"messageId":"<[email protected]>"}
. - Notice that the order number and delivery date will be missing from the email received. The reason is that using the API reference interface, you cannot specify rich JSON body parameters like the
params
to provideDATE
andORDER
, so you'll have to use the code snippets and test using cURL or using other integrations. Go to next section for more details. - If the parameters you entered are wrong, you'll get a 400 error along with an error message and error code. Find the list of errors here.
API Reference limitations and rating
When you try an endpoint, you will make a real call to the API.
Make sure to check rate limits and your credits as the API reference will use them to make the requests (when sending an email or an sms for instance).Read more about Brevo sending email bandwidth and quota
Creating your API request using Brevo API clients:
The code snippets in the API Reference are only there for you to test your API quickly and in the language that suits you.
👍 For your integration, we recommend you use our API clients that will provide you with shortcuts and ease of integration.
Available API clients and their documentations: C#, Go, Java, Node JS, PHP, Python, Ruby.
Here are sample codes using the API clients. Before using then, follow the installation guide from each documentation linked above.
curl --request POST \
--url https://api.brevo.com/v3/smtp/email \
--header 'accept: application/json' \
--header 'api-key:YOUR_API_KEY' \
--header 'content-type: application/json' \
--data '{
"to":[
{
"email":"[email protected]",
"name":"John Doe"
}
],
"templateId":8,
"params":{
"name":"John",
"surname":"Doe"
},
"headers":{
"X-Mailin-custom":"custom_header_1:custom_value_1|custom_header_2:custom_value_2|custom_header_3:custom_value_3",
"charset":"iso-8859-1"
}
}'
curl --request POST \
--url https://api.sendinblue.com/v3/smtp/email \
--header 'accept: application/json' \
--header 'api-key:YOUR_API_KEY' \
--header 'content-type: application/json' \
--data '{
"sender":{
"name":"Sender Alex",
"email":"[email protected]"
},
"to":[
{
"email":"[email protected]",
"name":"John Doe"
}
],
"subject":"test mail",
"htmlContent":"<html><head></head><body><h1>Hello this is a test email from sib</h1></body></html>",
"headers":{
"X-Mailin-custom":"custom_header_1:custom_value_1|custom_header_2:custom_value_2|custom_header_3:custom_value_3",
"charset":"iso-8859-1"
}
}'
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Configure API key authorization: api-key
$config = SendinBlue\Client\Configuration::getDefaultConfiguration()->setApiKey('api-key', 'YOUR_API_KEY');
// Uncomment below line to configure authorization using: partner-key
// $config = SendinBlue\Client\Configuration::getDefaultConfiguration()->setApiKey('partner-key', 'YOUR_API_KEY');
$apiInstance = new SendinBlue\Client\Api\TransactionalEmailsApi(
// If you want use custom http client, pass your client which implements `GuzzleHttp\ClientInterface`.
// This is optional, `GuzzleHttp\Client` will be used as default.
new GuzzleHttp\Client(),
$config
);
$sendSmtpEmail = new \SendinBlue\Client\Model\SendSmtpEmail(); // \SendinBlue\Client\Model\SendSmtpEmail | Values to send a transactional email
$sendSmtpEmail['to'] = array(array('email'=>'[email protected]', 'name'=>'John Doe'));
$sendSmtpEmail['templateId'] = 59;
$sendSmtpEmail['params'] = array('name'=>'John', 'surname'=>'Doe');
$sendSmtpEmail['headers'] = array('X-Mailin-custom'=>'custom_header_1:custom_value_1|custom_header_2:custom_value_2');
try {
$result = $apiInstance->sendTransacEmail($sendSmtpEmail);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling TransactionalEmailsApi->sendTransacEmail: ', $e->getMessage(), PHP_EOL;
}
?>
var SibApiV3Sdk = require('sib-api-v3-sdk');
var defaultClient = SibApiV3Sdk.ApiClient.instance;
// Configure API key authorization: api-key
var apiKey = defaultClient.authentications['api-key'];
apiKey.apiKey = 'YOUR_API_KEY';
// Uncomment below two lines to configure authorization using: partner-key
// var partnerKey = defaultClient.authentications['partner-key'];
// partnerKey.apiKey = 'YOUR API KEY';
var apiInstance = new SibApiV3Sdk.TransactionalEmailsApi();
var sendSmtpEmail = new SibApiV3Sdk.SendSmtpEmail(); // SendSmtpEmail | Values to send a transactional email
sendSmtpEmail = {
to: [{
email: '[email protected]',
name: 'John Doe'
}],
templateId: 59,
params: {
name: 'John',
surname: 'Doe'
},
headers: {
'X-Mailin-custom': 'custom_header_1:custom_value_1|custom_header_2:custom_value_2'
}
};
apiInstance.sendTransacEmail(sendSmtpEmail).then(function(data) {
console.log('API called successfully. Returned data: ' + data);
}, function(error) {
console.error(error);
});
# load the gem
require 'sib-api-v3-sdk'
# setup authorization
SibApiV3Sdk.configure do |config|
# Configure API key authorization: api-key
config.api_key['api-key'] = 'YOUR_API_KEY'
# Uncomment below line to configure API key authorization using: partner-key
# config.api_key['partner-key'] = 'YOUR API KEY'
end
api_instance = SibApiV3Sdk::TransactionalEmailsApi.new
send_smtp_email = SibApiV3Sdk::SendSmtpEmail.new # SendSmtpEmail | Values to send a transactional email
send_smtp_email = {
'to'=>[{
'email'=>'[email protected]',
'name'=>'John Doe'
}],
'templateId'=>59,
'params'=> {
'name'=>'John',
'surname'=>'Doe'
},
'headers'=> {
'X-Mailin-custom'=>'custom_header_1:custom_value_1|custom_header_2:custom_value_2'
}
};
begin
#Send a transactional email
result = api_instance.send_transac_email(send_smtp_email)
p result
rescue SibApiV3Sdk::ApiError => e
puts "Exception when calling TransactionalEmailsApi->send_transac_email: #{e}"
end
from __future__ import print_function
import time
import sib_api_v3_sdk
from sib_api_v3_sdk.rest import ApiException
from pprint import pprint
# Configure API key authorization: api-key
configuration = sib_api_v3_sdk.Configuration()
configuration.api_key['api-key'] = 'YOUR_API_KEY'
# Uncomment below lines to configure API key authorization using: partner-key
# configuration = sib_api_v3_sdk.Configuration()
# configuration.api_key['partner-key'] = 'YOUR_API_KEY'
# create an instance of the API class
api_instance = sib_api_v3_sdk.SMTPApi(sib_api_v3_sdk.ApiClient(configuration))
send_smtp_email = sib_api_v3_sdk.SendSmtpEmail(to=[{"email":"[email protected]","name":"John Doe"}], template_id=56, params={"name": "John", "surname": "Doe"}, headers={"X-Mailin-custom": "custom_header_1:custom_value_1|custom_header_2:custom_value_2|custom_header_3:custom_value_3", "charset": "iso-8859-1"}) # SendSmtpEmail | Values to send a transactional email
try:
# Send a transactional email
api_response = api_instance.send_transac_email(send_smtp_email)
pprint(api_response)
except ApiException as e:
print("Exception when calling SMTPApi->send_transac_email: %s\n" % e)
// This example is for Typescript-node
var SibApiV3Sdk = require('sib-api-v3-typescript');
var apiInstance = new SibApiV3Sdk.SMTPApi()
// Configure API key authorization: api-key
var apiKey = apiInstance.authentications['apiKey'];
apiKey.apiKey = "YOUR API KEY"
// Configure API key authorization: partner-key
var partnerKey = apiInstance.authentications['partnerKey'];
partnerKey.apiKey = "YOUR API KEY"
var sendSmtpEmail = {
to: [{
email: '[email protected]',
name: 'John Doe'
}],
templateId: 59,
params: {
name: 'John',
surname: 'Doe'
},
headers: {
'X-Mailin-custom': 'custom_header_1:custom_value_1|custom_header_2:custom_value_2'
}
};
apiInstance.sendTransacEmail(sendSmtpEmail).then(function(data) {
console.log('API called successfully. Returned data: ' + data);
}, function(error) {
console.error(error);
});
For each request, make sure to include the following headers:
api-key: xkeysib-xxxxxxxxxxxxxxxxx
content-type: application/json
accept: application/json
👍 Check your emails! Congratulations, you've just sent your first transactional email using Brevo API!
Tracking your transactional activity through Webhooks.
If you would like to additionally track the status of your message there's a number of events you can look after with webhooks. Here is the full list:
Sent , Delivered, Opened, Clicked, Soft Bounce, Hard Bounce, Invalid Email, Deferred, Complaint, Unsubscribed, Blocked, Error.
- We must create a webhook definition to which we will be posting all the event data. This can be easily done through the transactional webhooks page. . Here you need to specify the URL of the server which will be receiving the incoming data from Brevo. Also, you need to check which events you are interested to track.
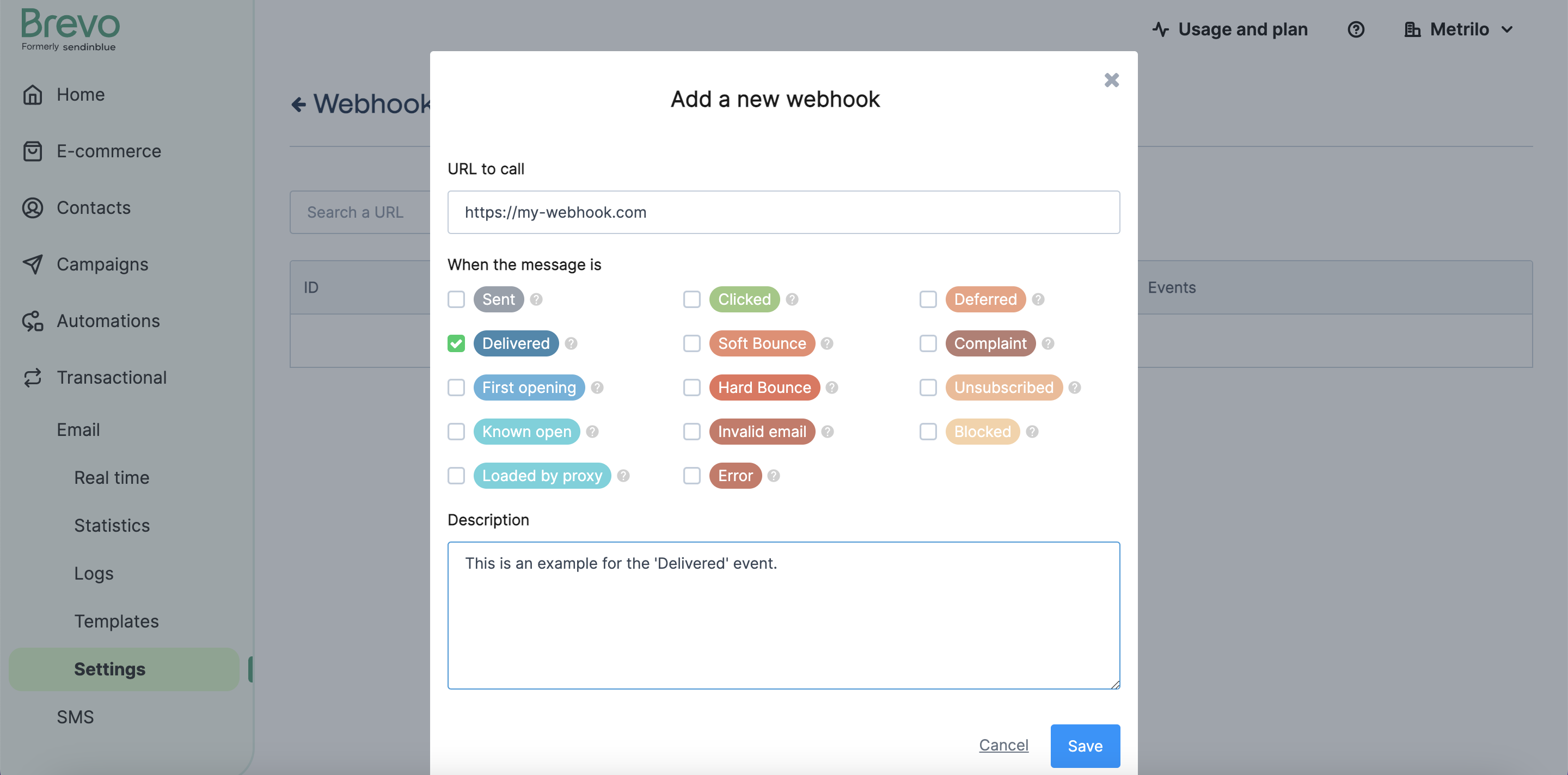
transactional webhooks page
Managing Webhooks
You can also do these actions straight from the API, if you prefer. The endpoints that will help you are Create a webhook and Update a webhook in case you want to change the webhook definition.
- We chose to track the
delivered
event in the previous step. Brevo will post the event specific data to your URL every time a transactional email is delivered to the recipient's inbox. Let's send another email:
curl --request POST \
--url https://api.brevo.com/v3/smtp/email \
--header 'accept: application/json' \
--header 'api-key: xkeysib-xxxxxxxxxxx' \
--header 'content-type: application/json' \
--data '{
"sender":{"email":"[email protected]"},
"to":[{"email":"[email protected]"}],
"replyTo":{"email":"[email protected]"},
"textContent":"This is a transactional email",
"subject":"Subject Line",
"tags":["myFirstTransactional"]
}'
Pro Tip
When sending a transactional email you can also pass the
tags
object to specify a custom flag or identifier which could help you query through the received events on your side.
- Once our message is delivered the following data will be posted to your URL. You can expect the same structure every time an event of the same kind is triggered.
{
"event": "delivered",
"email": "[email protected]",
"id": 26224,
"date": "YYYY-MM-DD HH:mm:ss",
"ts": 1598634509,
"message-id": "<[email protected]>",
"ts_event": 1598034509,
"subject": "Subject Line",
"tag": "[\"transactionalTag\"]",
"sending_ip": "185.41.28.109",
"ts_epoch": 1598634509223,
"tags": [
"myFirstTransactional"
]
}
Note: You can take a closer look to all the event specific payload in this help article.
Updated 7 months ago